Introduction
The manipulation and dealing with of date and time in programming are important for numerous functions. Python, being a flexible programming language, gives a strong DateTime module that simplifies working with dates and occasions. One essential operate inside the DateTime module is strptime(), which stands for “string parse time.” On this weblog submit, we’ll delve into the small print of the strptime() operate and discover how it may be a robust instrument for parsing strings into DateTime objects.
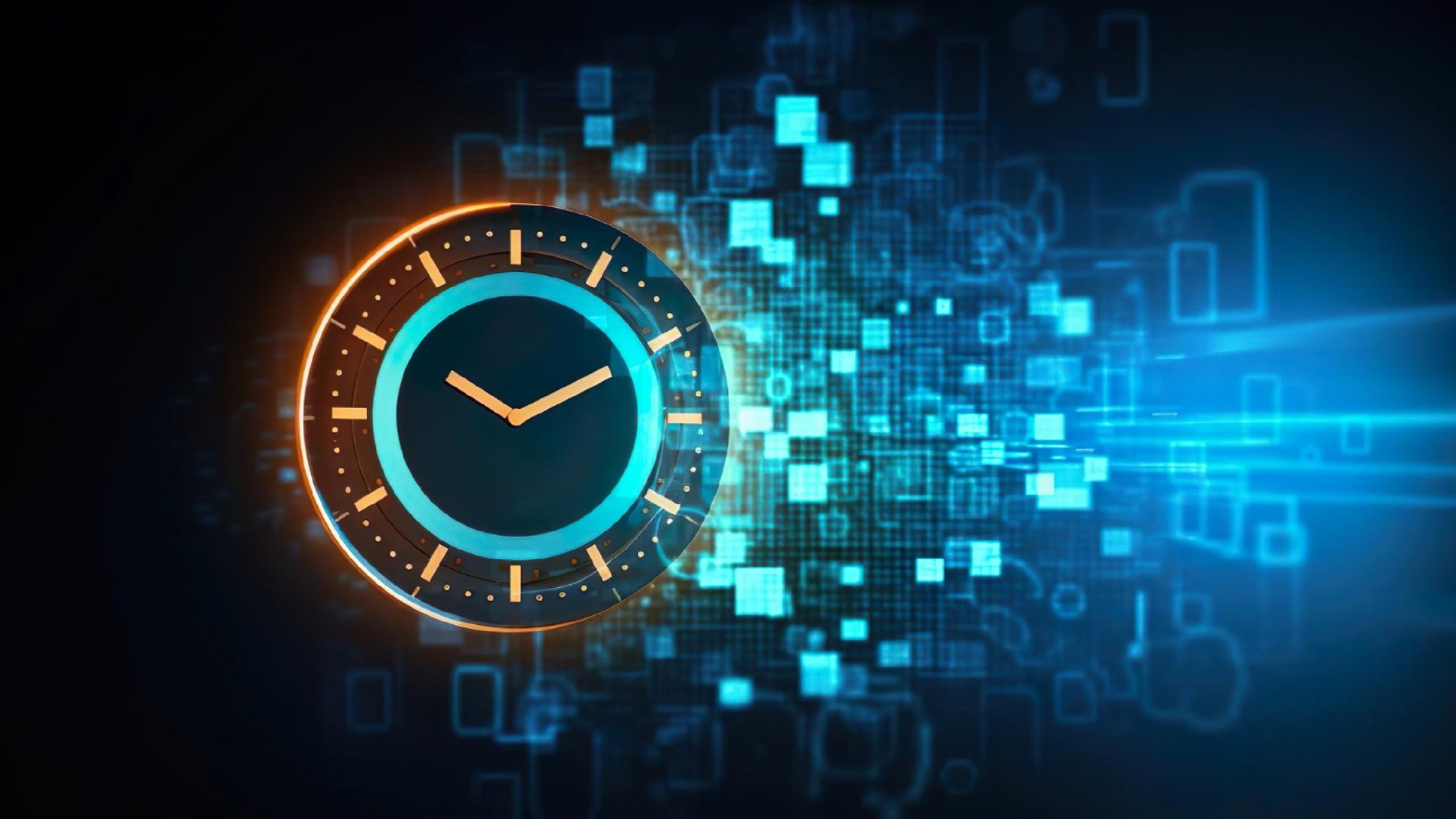
Understanding the `strptime()` Perform
The `strptime()` operate within the Python DateTime module is used to parse a string representing a date and time and convert it right into a DateTime object. It takes two arguments: the string to be parsed and the format of the string.
The string format is specified utilizing formatting directives, that are placeholders representing totally different date and time elements. These formatting directives are used to extract the related info from the string and create a DateTime object.
Formatting Directives for `strptime()`
The `strptime()` operate makes use of numerous formatting directives to specify the string format. Listed below are some generally used formatting directives:
1. `%Y` – 12 months: This directive represents the 12 months in 4 digits. For instance, `%Y` will parse the 12 months as ‘2022’.
2. `%m` – Month: This directive represents the month in two digits. For instance, `%m` will parse the month as ’01’ for January.
3. `%d` – Day: This directive represents the day in two digits. For instance, `%d` will parse the day as ’01’.
4. `%H` – Hour: This directive represents the hour in a 24-hour format. For instance, `%H` will parse the hour as ’13’ for 1 PM.
5. `%M` – Minute: This directive represents the minute in two digits. For instance, `%M` will parse the minute as ’30’.
6. `%S` – Second: This directive represents the second in two digits. For instance, `%S` will parse the second as ’45’.
7. `%A` – Weekday (Full identify): This directive represents the weekday’s full identify. For instance, `%A` will parse the weekday as ‘Monday’.
8. `%a` – Weekday (Abbreviation): This directive is used to signify the abbreviation of the weekday. For instance, `%a` will parse the weekday as ‘Mon’.
9. `%B` – Month (Full identify): This directive represents the month’s full identify. For instance, `%B` will parse the month as ‘January’.
10. `%b` – Month (Abbreviation): This directive represents the abbreviation of the month. For instance, `%b` will parse the month as ‘Jan’.
11. `%p` – AM/PM Indicator: This directive represents the AM/PM indicator. For instance, `%p` will parse the indicator as ‘AM’ or ‘PM’.
12. `%Z` – Timezone: This directive represents the timezone. For instance, `%Z` will parse the timezone as ‘UTC’ or ‘GMT’.
Examples of Utilizing `strptime()`
Changing a String to a Python DateTime Object
Suppose we’ve a string illustration of a date and time: ‘2022-01-01 13:30:45’. We are able to use the `strptime()` operate to transform this string right into a DateTime object as follows:
Code:
from datetime import datetime
date_string = '2022-01-01 13:30:45'
date_object = datetime.strptime(date_string, '%Y-%m-%d %H:%M:%S')
print(date_object)
Output:
2022-01-01 13:30:45
Dealing with Totally different Date Codecs
The `strptime()` operate can deal with totally different date codecs. Let’s say we’ve a string illustration of a date within the format ’01-Jan-2022′. We are able to use the `strptime()` operate with the suitable format directive to transform this string right into a DateTime object:
Code:
from datetime import datetime
date_string = '01-Jan-2022'
date_object = datetime.strptime(date_string, '%d-%b-%Y')
print(date_object)
Output:
2022-01-01 00:00:00
Parsing Timezones with `strptime()`
The `strptime()` operate may parse time zones. Let’s say we’ve a string illustration of a date and time with a timezone: ‘2022-01-01 13:30:45 UTC’. We are able to use the `strptime()` operate with the suitable format directive to transform this string right into a DateTime object:
Code:
from datetime import datetime
date_string = '2022-01-01 13:30:45 UTC'
date_object = datetime.strptime(date_string, '%Y-%m-%d %H:%M:%S %Z')
print(date_object)
Output:
2022-01-01 13:30:45+00:00
`ValueError: Unconverted Information Stays`
Typically, when utilizing the `strptime()` operate, it’s possible you’ll encounter a ValueError with the message “Unconverted knowledge stays”. This error happens when extra knowledge within the string isn’t transformed in accordance with the desired format. To repair this error, make sure the format matches the string precisely.
Code:
from datetime import datetime
date_string = '2022-01-01 13:30:45 ExtraData'
strive:
date_object = datetime.strptime(date_string, '%Y-%m-%d %H:%M:%S')
print(date_object)
besides ValueError as e:
print(f"Error: e")
Output:
Error: unconverted knowledge stays: ExtraData
`ValueError: time knowledge ‘…’ doesn’t match format ‘…’`
One other widespread error is the ValueError with the message “time knowledge ‘…’ doesn’t match format ‘…’”. This error happens when the string doesn’t match the desired format. Double-check the format and the string to make sure they’re appropriate.
Code:
from datetime import datetime
date_string = '01-01-2022'
strive:
date_object = datetime.strptime(date_string, '%Y-%m-%d')
print(date_object)
besides ValueError as e:
print(f"Error: e")
Output:
Error: time knowledge ’01-01-2022′ doesn’t match format ‘%Y-%m-%d’
Dealing with Invalid Dates or Instances
The `strptime()` operate doesn’t deal with invalid dates or occasions by default. When you move an invalid date or time, it should elevate a ValueError. You need to use exception dealing with with a try-except block to deal with invalid dates or occasions.
Code:
from datetime import datetime
date_string = '2022-02-30'
strive:
date_object = datetime.strptime(date_string, '%Y-%m-%d')
print(date_object)
besides ValueError as e:
print(f"Error: e")
Output:
Error: day is out of vary for month
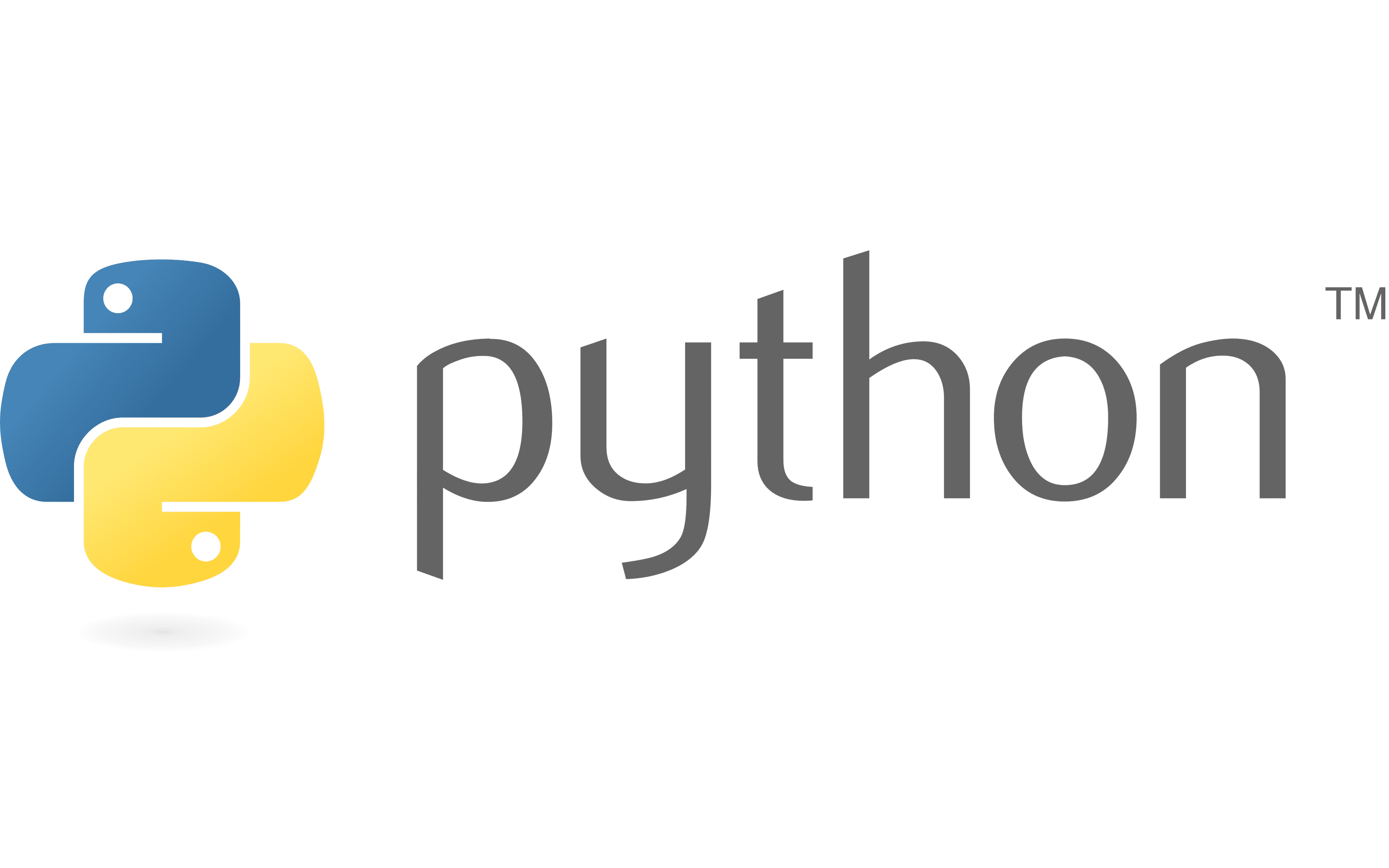
Finest Practices and Ideas for Utilizing `strptime()`
Specifying the Appropriate Format
When utilizing the `strptime()` operate, it’s essential to specify the right format for the string. Make sure that to match the formatting directives with the corresponding elements within the string. Failure to take action could lead to incorrect parsing or ValueError.
Dealing with Ambiguous Dates
Ambiguous dates, equivalent to ’01-02-2022′, might be interpreted otherwise relying on the date format. To keep away from ambiguity, utilizing unambiguous date codecs or offering extra context to make clear the date is really helpful.
Coping with Timezone Variations
When parsing strings with timezones, it’s important to contemplate the timezone variations. Be certain that the DateTime object is within the appropriate timezone or convert it to a desired timezone utilizing the suitable strategies.
Conclusion
The `strptime()` operate within the Python DateTime module is a robust instrument for changing string representations of dates and occasions into DateTime objects. By understanding the formatting directives and following greatest practices, you possibly can successfully parse and manipulate dates and occasions in your Python applications.
Able to embark on a journey to grasp Python and delve into the thrilling realms of Synthetic Intelligence and Machine Studying? Be part of our Licensed AI & ML BlackBelt Plus Program as we speak! Whether or not you’re a newbie keen to begin your programming journey or an skilled developer seeking to upskill, our complete program caters to all ability ranges. Acquire hands-on expertise, obtain skilled steering, and unlock the potential of Python within the dynamic fields of AI and ML. Don’t miss this chance to change into an authorized Python AI & ML BlackBelt – enroll now and take step one in direction of a rewarding and future-proof profession!